ggplot2’s facet options are a great way make small multiples, i.e. multiple plots of the same type in a panel or grid. In this post, we will learn how to control the border line in a plot made with facet_wrap() function in ggplot2.
First we will see how to remove the border lines in a facet using panel.border argument in theme() function. And then we will learn how to remove the spacings between the plots in the panel using “panel.spacing.x” argument to theme() function.
A Boxplot with facet_wrap
Let us get started by loading tidyverse and palmer penguins dataset for making a plot using facet_wrap(). As we all know ggplot2 makes a plot with grey background by default and here we change it to white background using theme_bw() option.
library(tidyverse) library(palmerpenguins) # set theme_bw() theme_set(theme_bw(18))
Here is how our data looks like.
penguins %>% head() ## # A tibble: 6 x 8 ## species island bill_length_mm bill_depth_mm flipper_length_… body_mass_g sex ## <fct> <fct> <dbl> <dbl> <int> <int> <fct> ## 1 Adelie Torge… 39.1 18.7 181 3750 male ## 2 Adelie Torge… 39.5 17.4 186 3800 fema… ## 3 Adelie Torge… 40.3 18 195 3250 fema… ## 4 Adelie Torge… 36.7 19.3 193 3450 fema… ## 5 Adelie Torge… 39.3 20.6 190 3650 male ## 6 Adelie Torge… 38.9 17.8 181 3625 fema… ## # … with 1 more variable: year <int>
We will make boxplot between sex and bill length from palmer penguins data and use facet_wrap() to make the boxplot for all species in the dataset.
penguins %>% ggplot(aes(x=sex, y=bill_length_mm, color=sex))+ geom_boxplot(outlier.shape = NA, width=0.5)+ geom_jitter(width=0.15, alpha=0.5)+ facet_wrap(~species)+ theme(legend.position="none")
Our grouped boxoplot made with face_wrap() looks like this, where each panel correspond to a specific species.
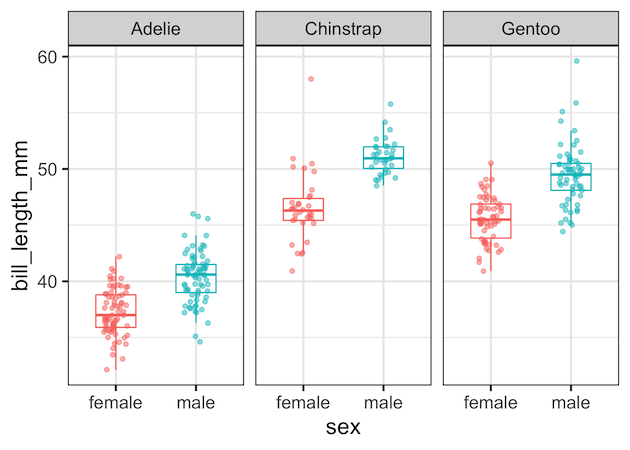
How to Remove Panel Border Lines in a facet in ggplot2?
Note that each panel has a outline box and we can control the panel border using panel.border argumnet in theme() function. To remove the panel border, we set “panel.border=element_blank()”.
penguins %>% ggplot(aes(x=sex, y=bill_length_mm, color=sex))+ geom_boxplot(outlier.shape = NA, width=0.5)+ geom_jitter(width=0.15, alpha=0.5)+ facet_wrap(~species)+ theme(legend.position="none", panel.border = element_blank())
Now we get a face plot without any panel borders.
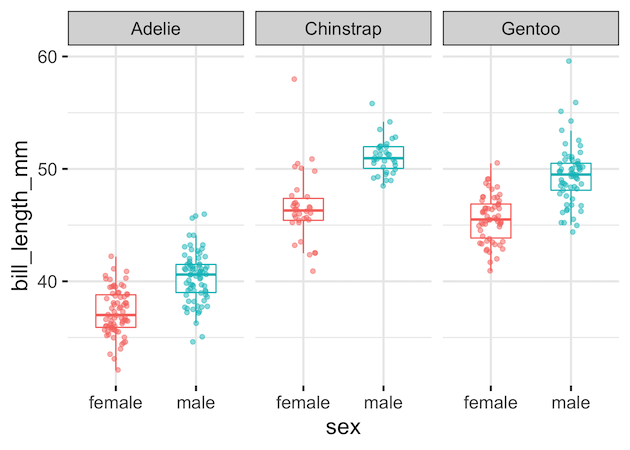
How to Remove Spacing between Panels in a facet in ggplot2?
Sometimes you might want to remove the empty spacings between each panel in a facet plot. We can customize the spacing between panels using “panel.spacing.x” argument to theme() function again.
In this example, we remove the spacing between panels by setting ‘panel.spacing.x = unit(0,”line”)’.
penguins %>% ggplot(aes(x=sex, y=bill_length_mm, color=sex))+ geom_boxplot(outlier.shape = NA, width=0.5)+ geom_jitter(width=0.15, alpha=0.5)+ facet_wrap(~species)+ theme(legend.position="none", panel.border = element_blank(), panel.spacing.x = unit(0,"line"))
After removing the panel spacing our plot looks like grouped boxplot with labels for each group, in this case for each species.
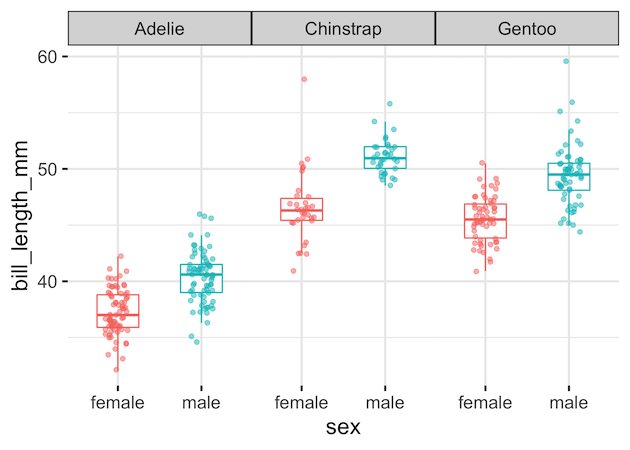