Barplots are great to visualize a quantitative value corresponding to categorical variables. Often it is more meaningful to make barplot sorted by the quantitative value corresponding to the bars.
In this post, we will see examples of making barplot using Seaborn and sorting the bars of barplot.
Let us load the packages needed to make barplots in Python.
import seaborn as sns import matplotlib.pyplot as plt import pandas as pd import numpy as np
We will create data to make the barplots. Here we create salary and education data inspired by average salary information from Stack Overflow developer survey.
education=["Bachelor's", "Less than Bachelor's","Master's","PhD","Professional"] salary = [110000,105000,126000,144200,95967]
Let us create Pandas dataframe with the data.
df = pd.DataFrame({"Education":education, "Salary":salary}) df Education Salary 0 Bachelor's 110000 1 Less than Bachelor's 105000 2 Master's 126000 3 PhD 144200 4 Professional 95967
In this example, we have the quantitative values corresponding to the bars. Before showing how to sort a barplot, we will first make a simple barplot using Seaborn’s barplot() function.
To make a barplot, we need to specify x and y-axis variables for the barplot as arguments to the Seaborn function. .
plt.figure(figsize=(10,6)) # make barplot sns.barplot(x='Education', y="Salary", data=df) # set labels plt.xlabel("Education", size=15) plt.ylabel("Salary in US Dollars", size=15) plt.title("Bar plot with Seaborn", size=18) plt.tight_layout() plt.savefig("barplot_Seaborn_Python.png", dpi=100)
Here, we have further customized the simple barplot by making the axis label text bigger and easier to read.
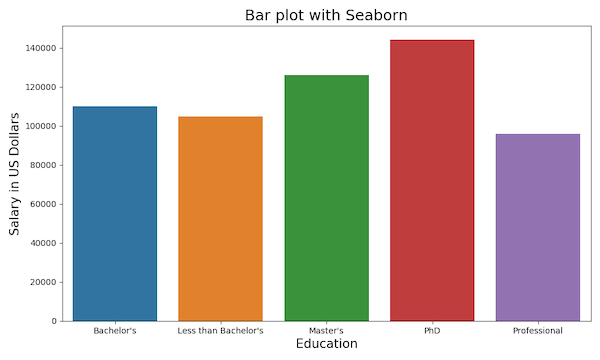
Sort Bars in Barplot in Ascending Order in Python
Let us move on to sort the bars in barplot. We can use “order” argument in Seaborn’s barplot() function to sort the bars. To the order argument, we need to provide the x-axis variable in the order we want to plot. Here we find the order of the x-axis variable using sort_values() function in Pandas. Basically, we sort the data frame by “Salary” using sort_values() and getting the Education values.
plt.figure(figsize=(10,6)) # make barplot and sort bars sns.barplot(x='Education', y="Salary", data=df, order=df.sort_values('Salary').Education) # set labels plt.xlabel("Education", size=15) plt.ylabel("Salary in US Dollars", size=15) plt.title("Sort Bars in Barplot in Ascending Order", size=18) plt.tight_layout() plt.savefig("sort_bars_in_barplot_ascending_order_Seaborn_Python.png", dpi=100)
We have the barplot with ordered bars. By default, sort_values() sorts in ascending order and we get the bars sorted in ascending order.

Sort Bars in Barplot in Descending Order in Python
Now let us see an example of sorting bars in barplot in descending order. We need to specify “ascending = False” inside sort_values() function while using “order” argument.
plt.figure(figsize=(10,6)) # make barplot and sort bars in descending order sns.barplot(x='Education', y="Salary", data=df, order=df.sort_values('Salary',ascending = False).Education) # set labels plt.xlabel("Education", size=15) plt.ylabel("Salary in US Dollars", size=15) plt.title("Sort Bars in Barplot in Descending Order", size=18) plt.tight_layout() plt.savefig("sort_bars_in_barplot_descending_order_Seaborn_Python.png", dpi=100)
Hurray!..Now we get bars sorted in descending order with Seaborn.
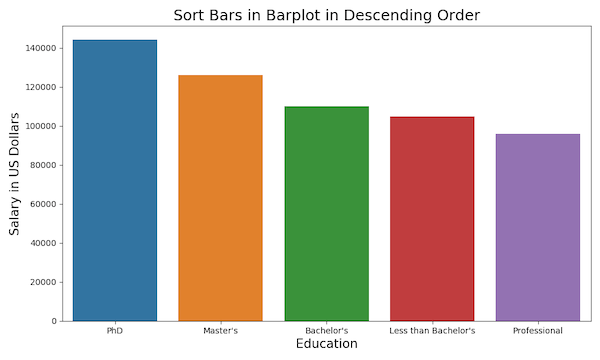